1. 파일 입출력
파이썬에서도 로컬 파일의 데이터를 읽거나 쓰는 작업을 할 수 있다. 텍스트 파일, 바이너리 파일, CSV 파일, JSON 파일 등을 읽고 쓰는 등 다양한 용도로 사용된다.
1-1. 파일 열기
파일을 열 땐 opne 함수를 사용한다.
f = open("파일명", "모드")
파일명: 열고자 하는 파일의 이름이나 경로
모드: 파일을 어떻게 열 것인지를 지정
r: 읽기 모드 (기본값)
w: 쓰기 모드 (파일이 있으면 덮어쓰기)
a: 추가 모드 (파일의 끝에 내용을 추가)
b: 바이너리 모드 (텍스트가 아닌 바이너리 데이터를 읽고/쓸 때 사용)
t: 텍스트 모드
+: 읽기와 쓰기 모드
1-2. 파일 쓰기
f = open('example.txt','wt' ) # 텍스트 파일을 만드는 것이기 때문에 출력 # 'wt'에서 t를 생략하고 'w'로 작성 가능
f.write('Hello 파이썬!\n')
f.close() # 파일을 닫아야 저장이 완료됨
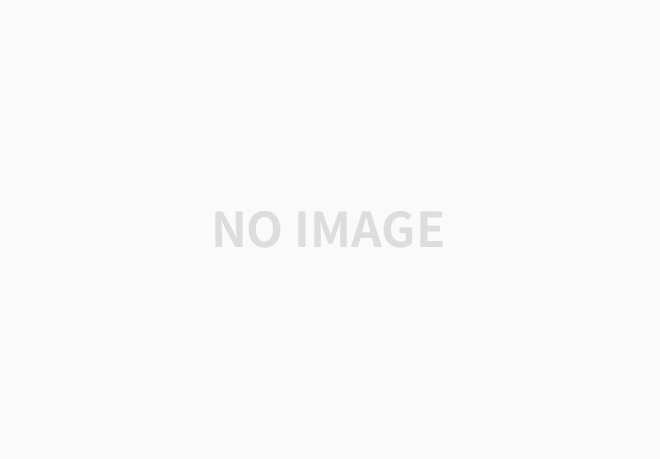
f = open('example.txt','wt' ) # 텍스트 파일을 만드는 것이기 때문에 출력 # 'wt'에서 t를 생략하고 'w'로 작성 가능
f.write('Hello 파이썬!\n')
f.close() # 파일을 닫아야 저장이 완료됨
f = open('example2.txt', 'w')
f.writelines(['라인1\n', '라인2\n', '라인3\n'])
f.close()
f = open('example3.txt', 'w')
for i in range(10):
f.write('파일 쓰기 테스트: ' + str(i) + '\n')
f.close()
print('example3.txt 파일에 쓰기 완료')
1-3. 파일 읽기
f = open('example.txt', 'rt')
data = f.read()
print(data)
f.close() # 안 닫아도 문제가 없지만 파일이 열려 있으면 다음에 읽을 때 문제가 발생할 수 있음
f = open('example2.txt', 'rt')
data = f.read() # 매개변수가 없을 경우 여러 줄을 한꺼번에 읽어옴
print(data)
f.close()
f = open('example2.txt', 'rt')
data = f.read(3) # 매개변수 만큼 읽어옴 즉 여기선 유니코드로 작성된 세 글자
print(data)
f.close()
f = open('example2.txt', 'rt')
data = f.read(5)
print(data)
f.close()
f = open('example3.txt', 'rt')
while True:
data = f.read(5)
if not data:
break
print(data, end = '')
f.close()
2. with 문 사용하기
- 파일을 보다 안전하고 깔끔하게 다루는 방법
- 컨텍스트 매니저를 사용하여 파일을 열고 파일 작업이 끝나면 자동으로 파일을 닫아주는 역할
- 파일을 열고 나서 오류가 발생하더라도 파일을 자동으로 닫아줌
- close() 호출하지 않아도 되므로 코드가 간단하고 깔끔해짐
with open('word.txt', 'w') as f:
while True:
data = input('단어를 입력하세요: ')
if data.lower() == 'quit':
break
f.write(data + '\n')
with open('example3.txt', 'rt') as f:
while True:
data = f.read(5)
if not data:
break
print(data, end = '')
with open('word.txt', 'r') as f:
lines = []
while True:
line = f.readline()
if not line:
break
print(line, end='')
lines.append(line.strip())
print(lines)
with open('word.txt', 'r') as f:
lines = f.readlines()
print(lines)
li = []
for i in lines:
li.append(i.replace('\n', ''))
print(li)
3. 예외 처리와 함께 사용하기
try:
with open('nofile.txt', 'r') as f:
data = f.read()
print(data)
except FileNotFoundError:
print('파일이 존재하지 않습니다')
'파이썬 > 파이썬 기초 문법' 카테고리의 다른 글
파이썬 넘파이 (0) | 2025.01.20 |
---|---|
파이썬 모듈 (2) | 2024.10.20 |
파이썬의 예외 처리 (0) | 2024.10.20 |
파이썬 스페셜 메서드 (4) | 2024.10.19 |
파이썬 상속 (2) | 2024.10.19 |